What is Object Oriented Programming?
Wondering What is Object-Oriented Programming.
OOP, is a popular programming paradigm that bundles data and methods together into structured units called 'objects'.
These objects represent and manipulate real-world entities, making your code more intuitive and modular.
OOP revolves around four key principles – encapsulation (grouping related data and functions), inheritance (deriving new classes from existing ones), polymorphism (single interface, multiple implementations), and abstraction (hiding complexity and displaying only necessary features).
By using OOP, developers can write more understandable, flexible, and maintainable code, making it a go-to approach for many complex software applications.
Concepts and Principles of OOPS
In this section, we're delving into the essential concepts and principles that underpin object-oriented programming, shaping its paradigm and defining its approach to structuring software applications.
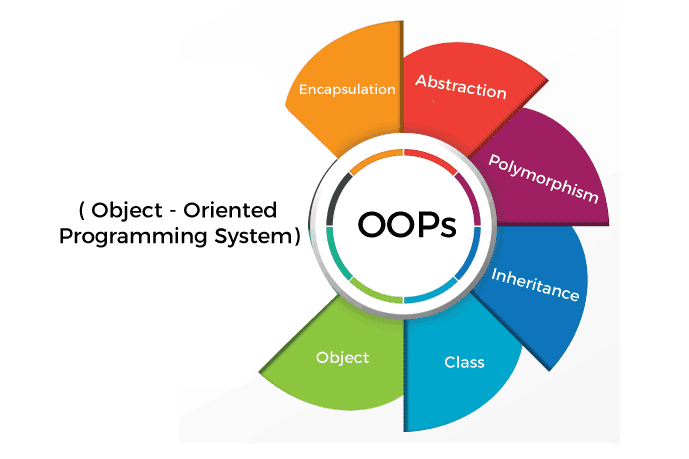
Encapsulation
Encapsulation allows us to bundle data and the methods that operate on this data within a single unit, known as an object.
It shields the object's internal state from outside interference only allowing interaction through provided methods.
Inheritance
Inheritance is a principle that lets new classes adopt properties and methods from existing classes.
It promotes code reusability and gives structure to code, leading to clearer and more organized software applications.
Polymorphism
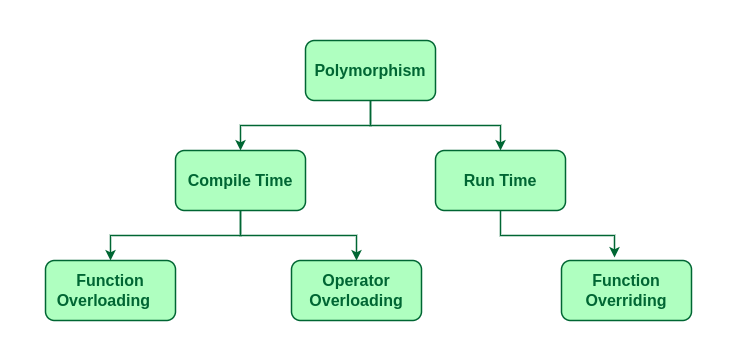
Polymorphism enables one interface to represent a variety of forms.
It allows us to use a single method in different ways for different input data types, leading to simpler, cleaner, and more efficient code.
Abstraction
Abstraction involves highlighting the essential features of a concept while ignoring irrelevant details.
It enables hiding complex implementations behind simple interfaces, enhancing usability and reducing complexity.
Class and Object
The concept of classes and objects is fundamental to OOP.
A class acts as a blueprint, and objects are instances of the class, containing properties (data) and methods (functions), enabling real-life modeling in software applications.
Constructor and Destructor
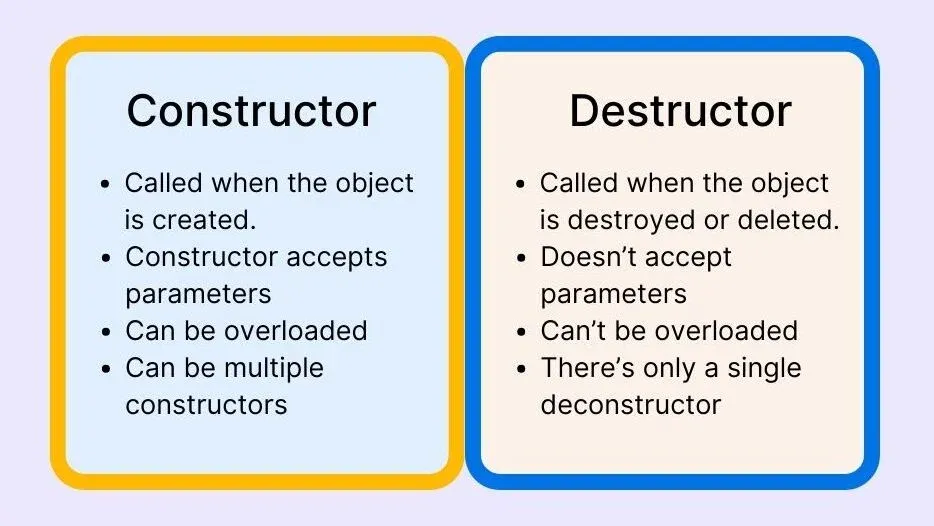
A constructor is a special method invoked at object creation, while a destructor is called when the object is no longer necessary.
They allow control over how an object is initialized and finalized, ensuring proper resource management.
Method Overloading and Overriding
Method overloading enables defining multiple methods with the same name but different parameters, increasing functionality.
Method overriding allows a subclass method to provide a different implementation than its superclass method, enhancing flexibility.
Association, Aggregation, and Composition
These three principles define relationships between classes.
Association represents a broad connection, aggregation entails a "has-a" relationship, and composition implies that one class owns another, establishing a whole-part hierarchy, facilitating complex software designs.
Interfaces and Packages
Interfaces define a contract for classes to follow, ensuring a consistent method of communication between objects.
Packages help in organizing related classes and interfaces together, encouraging modularity and cleaner code management.
Exception Handling
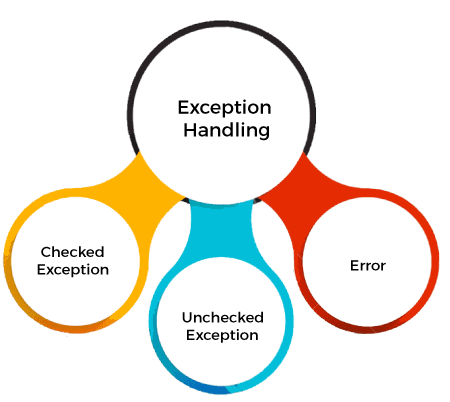
Exception handling in OOP allows for the detection and management of errors during execution, promoting robust and fault-resistant software applications.
Object-Oriented Programming Design Patterns
Design patterns are reusable solutions to common problems encountered in software design. Here are a few notable Object-Oriented Programming design patterns:
- Singleton: Ensures that a class has only one instance, with a global access point to that instance, optimizing resource usage and providing centralized control.
- Factory: Defines a method that creates objects, allowing subclasses to decide which class to instantiate, promoting a decoupled design and enhancing extensibility.
- Adapter: Converts the interface of a class into another interface expected by the client code, enabling the compatibility of classes with different interfaces, without modifying their source code.
- Observer: Establishes a one-to-many relationship between objects, wherein a change in one object's state results in automatic notification and updating of the related objects.
- Decorator: Enables adding new functionalities to an existing object dynamically, without altering its structure, enhancing an object's behavior without affecting other instances of the same class.
- Strategy: Encapsulates interchangeable algorithms within a family of algorithms, allowing the client to choose and change the algorithm at runtime based on their needs.
- Command: Encapsulates a request as an object, enabling parameterizing and queuing requests and promoting a decoupled design.
Understanding and utilizing these design patterns can improve the organization and efficiency of code in Object-Oriented Programming languages.
What is Object-Oriented Programming vs. Procedural Oriented Programming
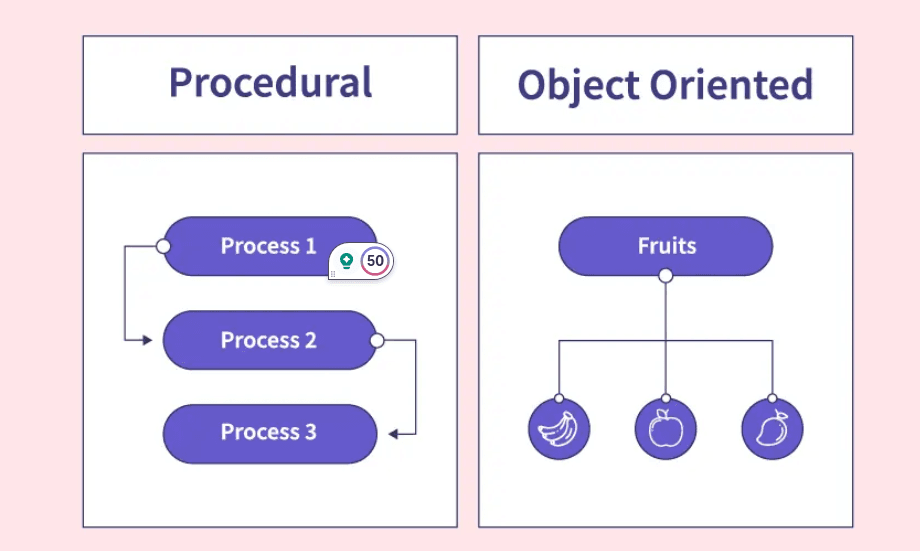
In this section, we'll compare Object-Oriented Programming (OOP) and Procedural Oriented Programming (POP), highlighting their differences and applications.
Programming Paradigms
OOP and POP are different programming paradigms that dictate how software is designed, organized, and coded.
OOP focuses on the organization of data and operations around objects, while POP primarily revolves around designing sequential procedures to solve problems.
Data and Functions
In OOP, code is organized into classes that embody both data and functions, or methods, that operate on the data.
This structure encourages the encapsulation of data and methods within objects, promoting modularity and maintainability.
On the other hand, POP involves the separation of data from functions, which leads to a more linear, step-by-step approach.
Code Reusability
OOP supports code reusability through inheritance, enabling you to create new classes derived from existing ones. This enhances efficiency and reduces redundancy.
Conversely, POP lacks inherent support for code reuse, which can lead to repetition and increased code complexity.
Ease of Maintenance and Modification
OOP's modular architecture simplifies maintenance and modification, as change in one object doesn't necessarily affect other objects in the system.
In contrast, POP's global data access and interdependent procedures can make modifications more cumbersome and error-prone.
Applications
While both paradigms have their place in software development, OOP is better suited for large, complex systems that require code scalability, maintenance, and reusability.
POP is better suited for smaller, highly focused programs with specific, well-defined tasks.
Examples of Object-Oriented Programming Languages
In this section, we'll discuss notable examples of Object-Oriented Programming (OOP) languages, each of which offers unique features and advantages to developers.
- Java: Perhaps one of the most ubiquitous OOP languages, Java's "write once, run anywhere" philosophy, robust frameworks, and extensive libraries have made it a staple in enterprise development, web applications, and even Android app development.
- C++: Born from the C language, C++ added classes and object-oriented principles to its predecessor's efficiency and low-level capabilities. It's often preferred for system/software development, game development, driver and firmware coding due to its powerful and versatile nature.
- Python: Known for its simplicity and readability, Python supports OOP, allowing for modular and reusable code. This versatility and user-friendly syntax have made it popular for web development, data analysis, AI, and machine learning.
- C#: C# is an OOP language developed by Microsoft, primarily used in developing Windows desktop applications and games with the Unity game engine. Its seamless integration with .NET framework makes it ideal for Microsoft-based development environments.
- Ruby: Ruby is praised for its elegance and readability. It's a fully object-oriented language, meaning everything in Ruby is an object, even primitives like numbers. It's also known for its Ruby on Rails framework, widely used in web development.
Each of these OOP languages has its own strengths and usage scenarios. The best one depends on the specific project requirements, platform, team expertise, and the development environment.
Advantages of Object-Oriented Programming
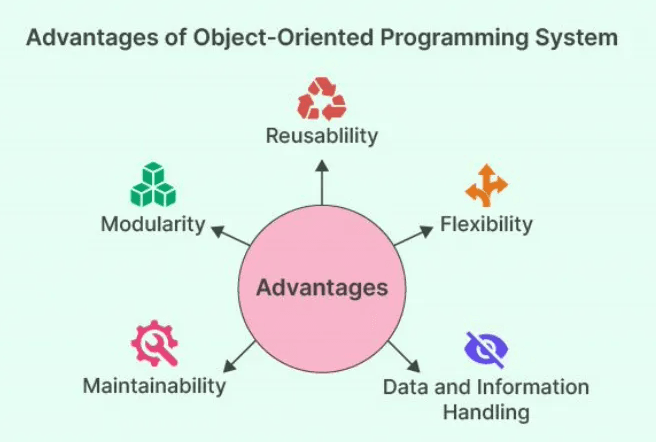
In this section, we'll examine the key benefits of adopting object-oriented programming, and how its principles bring efficiency and simplicity to the software development process.
Enhanced Security and Code Maintainability with Encapsulation
Encapsulation allows the bundling of data and the methods that operate on it within a single object. It hides the internal workings of an object, providing a clear interface for interaction.
This leads to increased security and simplified debugging and maintenance of code.
Unlocking Code Reusability and Organization with Inheritance
Inheritance enables new classes to adopt the properties and methods of existing ones, fostering code reusability and hierarchical organization.
This saves time and effort, as you can build upon existing code rather than recreating functionality, leading to efficient and manageable software development.
Flexibility and Simplification through Polymorphism
Polymorphism lets one interface represent multiple forms, which means you can use the same method name for different input data types.
This drives flexibility and streamlines the code, reducing the need for lengthy case statements or complex logic to handle diverse data types.
Boosting Code Readability and Reducing Complexity through Abstraction
Abstraction helps to focus on essential features while ignoring extraneous details.
By hiding complex implementations behind simple interfaces, it lowers the complexity of the code, making it more understandable and easier for developers to work.
Encouraging Modularity and Clarity with Classes and Objects
Object-oriented programming relies on classes and objects, enabling the organization of code into modules.
This promotes clarity, as these modules represent real-life entities, allowing developers to quickly grasp the relationships and structure of the application.
Frequently Asked Questions (FAQs)
What is the difference between a class and an object in OOP?
A class is a blueprint or template that defines the structure and behavior of objects.
An object, on the other hand, is an instance of a class, representing a specific entity with its own set of attributes and behaviors.
What is Object Oriented language?
Object-oriented languages (like Python, Java) are designed specifically for OOP.
These languages have features like classes (blueprints for objects) and inheritance (creating new objects from existing ones), making OOP development smooth
What are the main pillars of object-oriented programming?
The main pillars of object-oriented programming are:
- Abstraction
- Encapsulation
- Inheritance
- Polymorphism
What is the difference between method overloading and method overriding in OOP?
Method overloading is when multiple methods in a class have the same name but different parameters.
Method overriding, on the other hand, is when a subclass provides a different implementation of a method that is already defined in its superclass.
How does object-oriented programming enhance code maintenance and scalability?
Object-oriented programming enhances code maintenance and scalability by providing a modular and organized approach to code development.
Objects and classes allow for easy separation of concerns, making it easier to update, debug, and expand the codebase without affecting other parts of the program.
What is Object Oriented and Can you provide an example of how OOP is used in real-world applications?
Simply put, object-oriented refers to anything related to OOP. It's a way of thinking about programming that emphasizes objects and their interactions.
This approach helps create complex and well-organized software.
Sure! One example is the banking system.
Each customer can be represented as an object with their own account details and transactions. The account object can have methods for depositing, withdrawing, and checking the account balance.
Inheritance can be used to create specialized account types like savings accounts or checking accounts with additional behaviors.